Virtual Background enables users to blur their background, or replace it with a solid color or an image. This feature is applicable to scenarios such as online conferences, online classes, and live streaming. It helps protect personal privacy and reduces audience distraction.
Virtual Background offers the following options:
Feature | Example |
---|
Blurred background and image background | 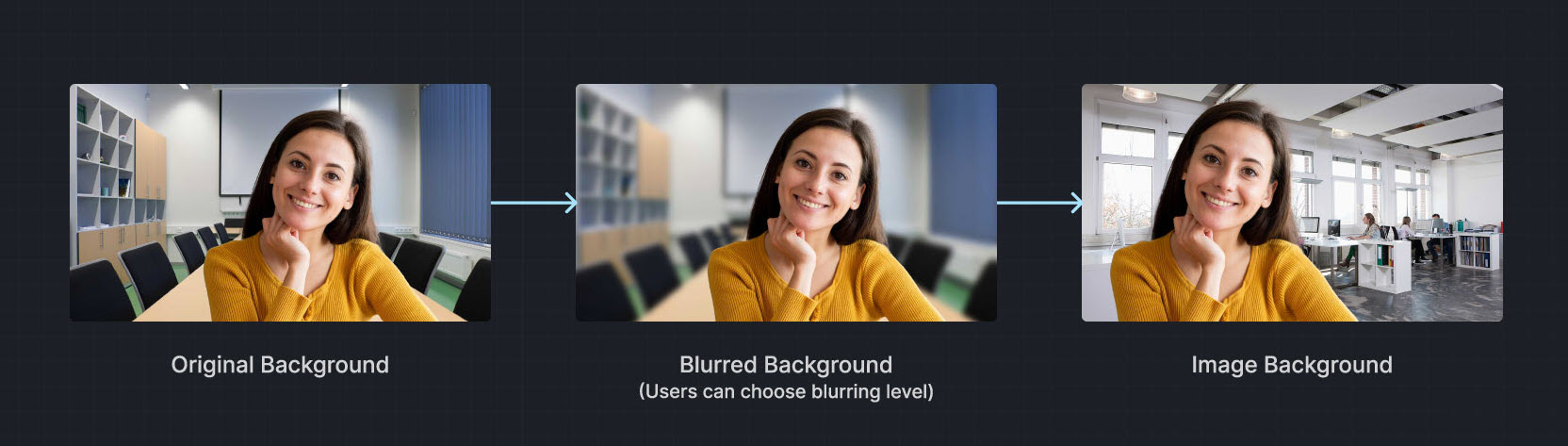 |
Video/Animated background | |
Portrait-in-picture | Allows the presenter to use slides as the virtual background while superimposing their video. The effect is similar to a weather news cast on television, preventing interruptions during a layout toggle. |
Want to test Virtual Background? Try the online demo.
Ensure that you have implemented the SDK quickstart in your project.
This section shows you how to add a virtual background to the local video.
To avoid performance degradation or unavailable features when enabling Virtual Background on low-end devices, check whether the device supports the feature.
_5boolean isFeatureAvailable() {
_5 return agoraEngine.isFeatureAvailableOnDevice(
_5 Constants.FEATURE_VIDEO_VIRTUAL_BACKGROUND
To blur the video background, use the following code:
_14void setBlurBackground() {
_14 VirtualBackgroundSource virtualBackgroundSource = new VirtualBackgroundSource();
_14 virtualBackgroundSource.setBackgroundSourceType(VirtualBackgroundSource.BACKGROUND_BLUR);
_14 virtualBackgroundSource.setBlurDegree(VirtualBackgroundSource.BLUR_DEGREE_MEDIUM);
_14 // Set processing properties for background
_14 SegmentationProperty segmentationProperty = new SegmentationProperty();
_14 segmentationProperty.setModelType(SegmentationProperty.SEG_MODEL_AI);
_14 // Use SEG_MODEL_GREEN if you have a green background
_14 segmentationProperty.setGreenCapacity(0.5f); // Accuracy for identifying green colors (range 0-1)
_14 // Enable or disable virtual background
_14 agoraEngine.enableVirtualBackground(true, virtualBackgroundSource, segmentationProperty);
To apply a solid color as the virtual background, use a hexadecimal color code. For example, 0x0000FF
for blue:
_14void setSolidBackground() {
_14 VirtualBackgroundSource virtualBackgroundSource = new VirtualBackgroundSource();
_14 virtualBackgroundSource.setBackgroundSourceType(VirtualBackgroundSource.BACKGROUND_COLOR);
_14 virtualBackgroundSource.setColor(0x0000FF);
_14 // Set processing properties for background
_14 SegmentationProperty segmentationProperty = new SegmentationProperty();
_14 segmentationProperty.setModelType(SegmentationProperty.SEG_MODEL_AI);
_14 // Use SEG_MODEL_GREEN if you have a green background
_14 segmentationProperty.setGreenCapacity(0.5f); // Accuracy for identifying green colors (range 0-1)
_14 // Enable or disable virtual background
_14 agoraEngine.enableVirtualBackground(true, virtualBackgroundSource, segmentationProperty);
To set a custom image as the virtual background, specify the absolute path to the image file.
_14void setImageBackground() {
_14 VirtualBackgroundSource virtualBackgroundSource = new VirtualBackgroundSource();
_14 virtualBackgroundSource.setBackgroundSourceType(VirtualBackgroundSource.BACKGROUND_IMG);
_14 virtualBackgroundSource.setSource("<absolute path to an image file>");
_14 // Set processing properties for background
_14 SegmentationProperty segmentationProperty = new SegmentationProperty();
_14 segmentationProperty.setModelType(SegmentationProperty.SEG_MODEL_AI);
_14 // Use SEG_MODEL_GREEN if you have a green background
_14 segmentationProperty.setGreenCapacity(0.5f); // Accuracy for identifying green colors (range 0-1)
_14 // Enable or disable virtual background
_14 agoraEngine.enableVirtualBackground(true, virtualBackgroundSource, segmentationProperty);
To disable the virtual background and revert to the original video state, use the following code:
_8void removeBackground() {
_8 // Disable virtual background
_8 agoraEngine.enableVirtualBackground(
_8 new VirtualBackgroundSource(),
_8 new SegmentationProperty()
This section contains content that completes the information in this page, or points you to documentation that explains other aspects to this product.